April 2, 2025
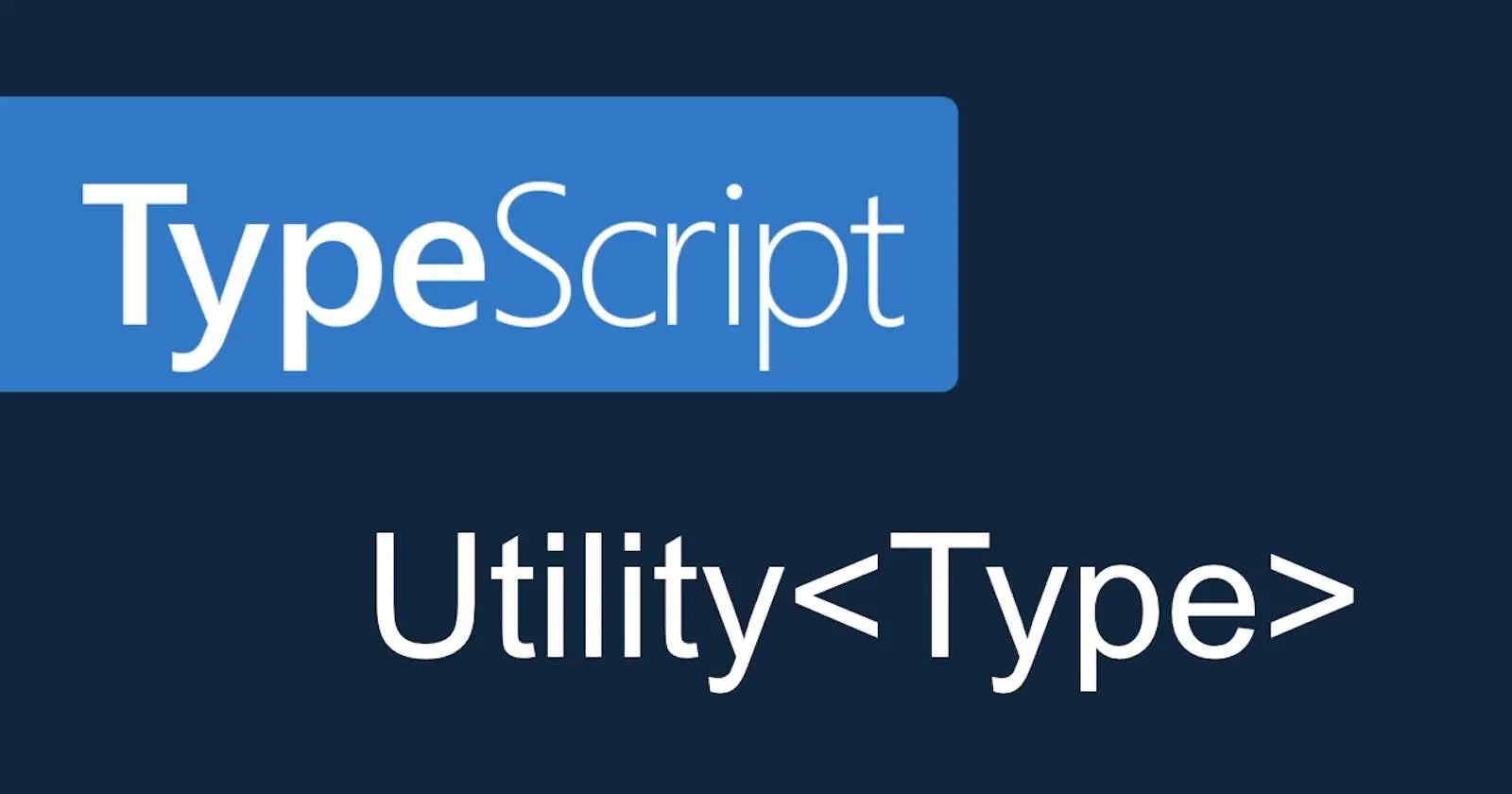
TypeScript Utility Types: Những loại hữu ích ít ai biết
Khám phá những TypeScript Utility Types ít ai biết nhưng cực kỳ hữu ích trong việc quản lý kiểu dữ liệu.
1. Partial<T>
: Biến tất cả thuộc tính của một đối tượng thành optional
Partial<T>
là một trong những loại hữu ích nhất khi làm việc với đối tượng. Nó giúp bạn dễ dàng thay đổi tất cả các thuộc tính của một loại đối tượng thành optional.
Ví dụ:
interface User {
name: string;
age: number;
}
const updateUser = (user: Partial<User>) => {
// Bạn chỉ cần truyền vào một số thuộc tính của user.
};
updateUser({ name: "John" });
Ở ví dụ trên, bạn có thể gọi updateUser
với một phần thông tin của User
, thay vì phải truyền vào đầy đủ tất cả thuộc tính.
2. Required<T>
: Biến tất cả thuộc tính của một đối tượng thành required
Ngược lại với Partial<T>
, Required<T>
làm tất cả thuộc tính trong một đối tượng trở thành required.
Ví dụ:
interface User {
name: string;
age?: number;
}
const user: Required<User> = {
name: "John",
age: 30, // Không thể bỏ qua age nữa
};
Trong ví dụ này, bạn sẽ không thể khởi tạo một đối tượng User
mà thiếu age
.
3. Pick<T, K>
: Chọn một số thuộc tính từ đối tượng
Pick<T, K>
giúp bạn tạo một kiểu mới từ một đối tượng đã có, chỉ với các thuộc tính được chỉ định.
Ví dụ:
interface User { name: string; age: number; email: string; } const contactInfo: Pick<User, "name" | "email"> = { name: "John", email: "john@example.com", };
Ở đây, Pick
giúp bạn chỉ lấy ra các thuộc tính name
và email
của User
, bỏ qua age
.
4. Omit<T, K>
: Loại bỏ một số thuộc tính khỏi đối tượng
Omit<T, K>
là loại ngược lại của Pick
. Nó cho phép bạn tạo một kiểu mới từ một đối tượng, nhưng loại bỏ các thuộc tính bạn không muốn.
Ví dụ:
interface User {
name: string;
age: number;
email: string;
}
const userWithoutEmail: Omit<User, "email"> = {
name: "John",
age: 30,
};
Ở ví dụ trên, Omit
giúp bạn tạo ra một đối tượng User
mà không có thuộc tính email
.
5. Record<K, T>
: Tạo đối tượng với key và value có kiểu xác định
Record<K, T>
cho phép bạn định nghĩa một đối tượng có các khóa là K
và giá trị là T
. Loại này rất hữu ích khi bạn cần một đối tượng với các khóa động.
Ví dụ:
type Role = "admin" | "user" | "guest"; const permissions: Record<Role, boolean> = { admin: true, user: false, guest: false, };
Trong ví dụ trên, Record
giúp tạo ra một đối tượng permissions
với các khóa là Role
và giá trị boolean.
6. Exclude<T, U>
: Loại bỏ các giá trị từ một kiểu
Exclude<T, U>
giúp bạn tạo một kiểu mới bằng cách loại bỏ các giá trị của U
từ T
. Loại này rất hữu ích khi bạn cần loại bỏ một số giá trị từ một kiểu hợp nhất (union type).
Ví dụ:
type Fruit = "apple" | "banana" | "orange"; type Citrus = Exclude<Fruit, "banana">; // Citrus chỉ còn lại "apple" và "orange"
Ở ví dụ trên, Exclude
giúp bạn loại bỏ "banana"
khỏi kiểu Fruit
.
7. NonNullable<T>
: Loại bỏ null
và undefined
NonNullable<T>
giúp loại bỏ các giá trị null
và undefined
khỏi kiểu T
. Đây là một cách tuyệt vời để đảm bảo rằng các giá trị luôn có giá trị hợp lệ.
Ví dụ:
type MyType = string | null | undefined; type MyNonNullableType = NonNullable<MyType>; // MyNonNullableType chỉ còn lại string
NonNullable
sẽ loại bỏ các giá trị null
và undefined
từ kiểu MyType
.
Tổng kết
Các Utility Types trong TypeScript giúp bạn viết mã sạch hơn, dễ duy trì hơn và tránh được các lỗi không đáng có khi làm việc với kiểu dữ liệu.
Partial<T>
: Biến tất cả thuộc tính thành optional.Required<T>
: Biến tất cả thuộc tính thành required.Pick<T, K>
: Lấy một số thuộc tính từ đối tượng.Omit<T, K>
: Loại bỏ một số thuộc tính khỏi đối tượng.Record<K, T>
: Tạo đối tượng với khóa và giá trị có kiểu xác định.Exclude<T, U>
: Loại bỏ các giá trị từ một kiểu.NonNullable<T>
: Loại bỏnull
vàundefined
.
Việc sử dụng đúng các Utility Types này sẽ giúp bạn quản lý kiểu dữ liệu hiệu quả hơn và tối ưu code của mình! 🚀
TypeScript Utility Types: Lesser-Known but Highly Useful Types
Discover lesser-known TypeScript Utility Types that are incredibly useful for managing data types.
1. Partial<T>
: Makes All Properties of an Object Optional
Partial<T>
is one of the most useful types when working with objects. It allows you to easily make all properties of a type optional.
Example:
interface User { name: string; age: number; } const updateUser = (user: Partial<User>) => { // You only need to pass in some properties of the user. }; updateUser({ name: "John" });
In the example above, you can call updateUser
with partial information of User
, rather than having to pass all properties.
2. Required<T>
: Makes All Properties of an Object Required
Opposite to Partial<T>
, Required<T>
makes all properties of an object required.
Example:
interface User {
name: string;
age?: number;
}
const user: Required<User> = {
name: "John",
age: 30, // The age cannot be omitted anymore
};
In this example, you cannot create a User
object without age
.
3. Pick<T, K>
: Select Some Properties from an Object
Pick<T, K>
allows you to create a new type from an existing object with only the specified properties.
Example:
interface User { name: string; age: number; email: string; } const contactInfo: Pick<User, "name" | "email"> = { name: "John", email: "john@example.com", };
Here, Pick
helps you extract only the name
and email
properties of User
, ignoring age
.
4. Omit<T, K>
: Remove Some Properties from an Object
Omit<T, K>
is the reverse of Pick
. It allows you to create a new type from an object but without certain properties.
Example:
interface User {
name: string;
age: number;
email: string;
}
const userWithoutEmail: Omit<User, "email"> = {
name: "John",
age: 30,
};
In this example, Omit
helps you create a User
object without the email
property.
5. Record<K, T>
: Create an Object with a Specific Key and Value Type
Record<K, T>
allows you to define an object with keys of type K
and values of type T
. This type is very useful when you need an object with dynamic keys.
Example:
type Role = "admin" | "user" | "guest"; const permissions: Record<Role, boolean> = { admin: true, user: false, guest: false, };
In this example, Record
helps create a permissions
object with keys from Role
and boolean values.
6. Exclude<T, U>
: Remove Values from a Type
Exclude<T, U>
helps you create a new type by removing values of type U
from T
. This type is useful when you need to remove specific values from a union type.
Example:
type Fruit = "apple" | "banana" | "orange"; type Citrus = Exclude<Fruit, "banana">; // Citrus only includes "apple" and "orange"
In this example, Exclude
helps you remove "banana"
from the Fruit
type.
7. NonNullable<T>
: Remove null
and undefined
NonNullable<T>
helps you remove null
and undefined
from a type. This is a great way to ensure that values always have valid values.
Example:
type MyType = string | null | undefined; type MyNonNullableType = NonNullable<MyType>; // MyNonNullableType only includes string
NonNullable
removes null
and undefined
from the MyType
type.
Summary
TypeScript Utility Types help you write cleaner, more maintainable code and avoid unnecessary errors when working with data types.
Partial<T>
: Makes all properties optional.Required<T>
: Makes all properties required.Pick<T, K>
: Selects some properties from an object.Omit<T, K>
: Removes some properties from an object.Record<K, T>
: Creates an object with specific key and value types.Exclude<T, U>
: Removes values from a type.NonNullable<T>
: Removesnull
andundefined
.
Using the correct utility types will help you manage your data types more efficiently and optimize your code! 🚀