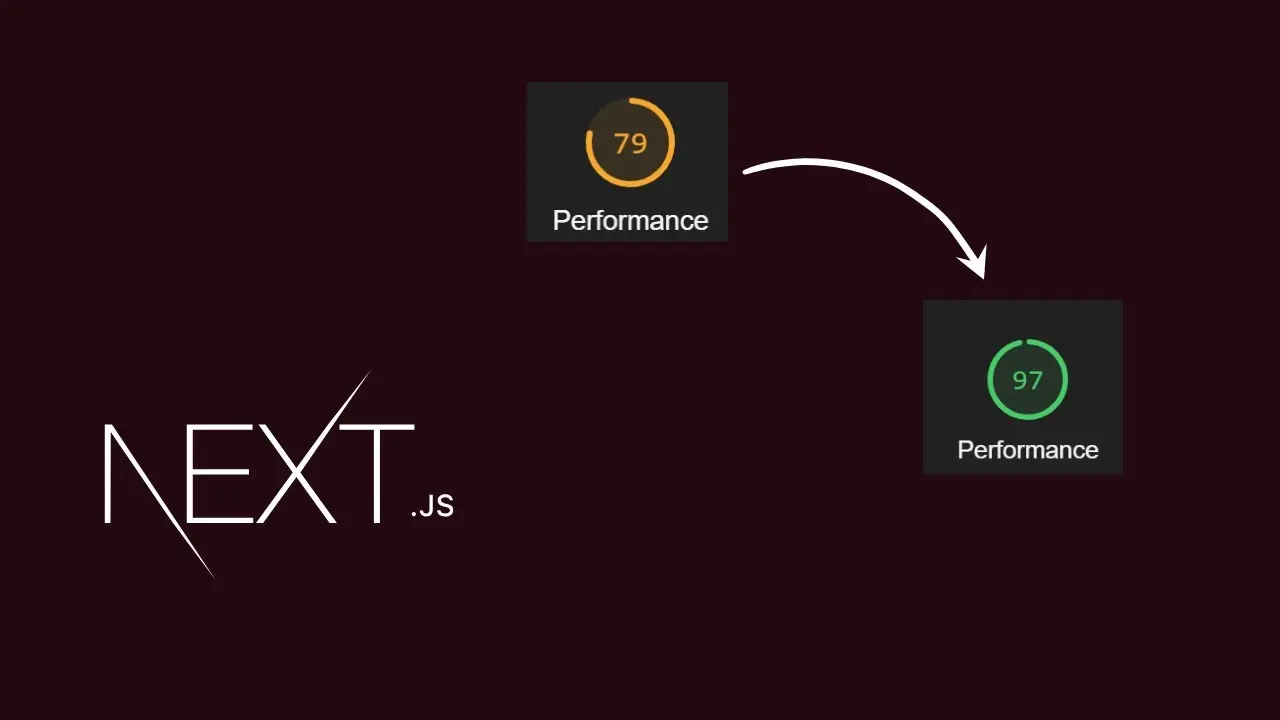
January 21, 2025
Tối ưu hiệu suất trong ứng dụng Next.js
Cách tối ưu hiệu suất cho ứng dụng Next.js để cải thiện trải nghiệm người dùng
Giới thiệu
Khi phát triển ứng dụng với Next.js, một trong những yếu tố quan trọng nhất là tối ưu hóa hiệu suất. Tối ưu hóa giúp cải thiện tốc độ tải trang, giảm độ trễ và mang lại trải nghiệm người dùng mượt mà hơn. Trong bài viết này, chúng ta sẽ khám phá những cách tối ưu hiệu suất cho ứng dụng Next.js từ việc tối ưu hóa hình ảnh, code splitting, cho đến sử dụng các tính năng tối ưu có sẵn trong Next.js.
1. Sử dụng Static Site Generation (SSG)
Một trong những tính năng mạnh mẽ nhất của Next.js là Static Site Generation (SSG). Với SSG, bạn có thể pre-render các trang ngay khi xây dựng ứng dụng, giúp trang web tải nhanh hơn vì nội dung đã có sẵn khi người dùng truy cập.
Cách sử dụng:
Trong Next.js, bạn có thể sử dụng getStaticProps
để fetch dữ liệu và pre-render trang. Điều này giúp trang web không cần phải gọi API mỗi khi người dùng truy cập.
// pages/index.tsx
import { GetStaticProps } from 'next';
export const getStaticProps: GetStaticProps = async () => {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: {
data
}
};
};
const Home = ({ data }) => {
return (
<div>
<h1>Welcome to the Optimized Next.js App!</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default Home;
2. Sử dụng Image Optimization
Một trong những yếu tố quan trọng trong việc tối ưu hóa hiệu suất là giảm kích thước hình ảnh. Next.js cung cấp một component next/image giúp tối ưu hóa hình ảnh tự động, bao gồm lazy loading, tự động điều chỉnh kích thước hình ảnh và tối ưu hóa định dạng.
Cách sử dụng:
import Image from 'next/image';
const MyComponent = () => (
<div>
<h1>Optimized Images</h1>
<Image
src="/images/example.jpg"
alt="Example Image"
width={500}
height={300}
quality={75}
/>
</div>
);
Với next/image, hình ảnh sẽ được tải nhanh hơn và tự động điều chỉnh cho phù hợp với kích thước màn hình của người dùng.
3. Code Splitting và Dynamic Import
Next.js hỗ trợ code splitting tự động, giúp giảm kích thước bundle JavaScript. Tuy nhiên, bạn cũng có thể sử dụng dynamic imports để tải các phần tử hoặc module chỉ khi cần thiết, điều này giúp giảm thời gian tải trang ban đầu.
Cách sử dụng:
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('./components/DynamicComponent'));
const Page = () => (
<div>
<h1>Dynamic Component Example</h1>
<DynamicComponent />
</div>
);
4. Tối ưu hóa CSS và JavaScript
Next.js hỗ trợ CSS-in-JS và có các tính năng như automatic static optimization giúp tự động loại bỏ CSS không sử dụng. Tuy nhiên, để tối ưu hơn, bạn có thể sử dụng công cụ như PurgeCSS hoặc Tailwind CSS để loại bỏ CSS không sử dụng trong sản phẩm cuối cùng.
Sử dụng Tailwind CSS để tối ưu hóa: Nếu bạn sử dụng Tailwind CSS, công cụ PurgeCSS sẽ tự động loại bỏ các lớp CSS không sử dụng khi build, giúp giảm kích thước CSS.
// tailwind.config.js module.exports = { purge: ['./pages/**/*.tsx', './components/**/*.tsx'], darkMode: false, theme: { extend: {} }, variants: { extend: {} }, plugins: [] };
5. Caching và CDN
Để tăng tốc độ tải trang, bạn có thể sử dụng caching và CDN để phân phối nội dung tĩnh như hình ảnh, CSS, và JavaScript. Next.js hỗ trợ tốt việc triển khai trên các dịch vụ như Vercel, Netlify, giúp phân phối ứng dụng qua mạng lưới các máy chủ gần người dùng nhất.
Cách sử dụng:
Bạn có thể cấu hình caching trong Next.js thông qua next.config.js để xác định các chiến lược caching phù hợp cho các tài nguyên của ứng dụng.
module.exports = { async headers() { return [ { source: '/(.*).(js|css|png|jpg|jpeg|svg|gif|webp|ico)', headers: [ { key: 'Cache-Control', value: 'public, max-age=31536000, immutable', }, ], }, ]; }, };
6. Cải thiện SEO với Server-Side Rendering (SSR)
Nếu ứng dụng của bạn cần phải có SEO tốt hơn, bạn có thể sử dụng Server-Side Rendering (SSR) với Next.js để render nội dung trên server trước khi gửi về client. Điều này giúp công cụ tìm kiếm dễ dàng index nội dung trang.
// pages/index.tsx import { GetServerSideProps } from 'next'; export const getServerSideProps: GetServerSideProps = async () => { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data } }; };
Kết luận
Tối ưu hóa hiệu suất trong Next.js không chỉ giúp cải thiện trải nghiệm người dùng mà còn góp phần vào việc tăng trưởng SEO và khả năng mở rộng ứng dụng. Bằng cách tận dụng các tính năng như SSG, tối ưu hóa hình ảnh, dynamic imports và caching, bạn có thể xây dựng các ứng dụng Next.js nhanh chóng và hiệu quả.
Optimizing Performance in Next.js Applications
How to optimize performance for Next.js applications to improve user experience
Introduction
When developing applications with Next.js, one of the most important factors is performance optimization. Optimization helps improve page load speed, reduce latency, and deliver a smoother user experience. In this article, we’ll explore various ways to optimize the performance of a Next.js application, from image optimization and code splitting to leveraging built-in Next.js features.
1. Use Static Site Generation (SSG)
One of Next.js’s most powerful features is Static Site Generation (SSG). With SSG, you can pre-render pages at build time, allowing your website to load faster since the content is already available when users visit.
How to use:
In Next.js, you can use getStaticProps
to fetch data and pre-render the page. This eliminates the need to call an API every time a user visits the page.
// pages/index.tsx
import { GetStaticProps } from 'next';
export const getStaticProps: GetStaticProps = async () => {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: {
data
}
};
};
const Home = ({ data }) => {
return (
<div>
<h1>Welcome to the Optimized Next.js App!</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default Home;
2. Use Image Optimization
An important aspect of performance optimization is reducing image sizes. Next.js provides the next/image component, which automatically optimizes images, including lazy loading, automatic resizing, and format optimization.
How to use:
import Image from 'next/image';
const MyComponent = () => (
<div>
<h1>Optimized Images</h1>
<Image
src="/images/example.jpg"
alt="Example Image"
width={500}
height={300}
quality={75}
/>
</div>
);
With next/image, images load faster and automatically adjust based on the user's screen size.
3. Code Splitting and Dynamic Import
Next.js supports automatic code splitting, which reduces the size of the JavaScript bundle. You can also use dynamic imports to load components or modules only when needed, helping to reduce initial page load time.
How to use:
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('./components/DynamicComponent'));
const Page = () => (
<div>
<h1>Dynamic Component Example</h1>
<DynamicComponent />
</div>
);
4. Optimize CSS and JavaScript
Next.js supports CSS-in-JS and features like automatic static optimization, which helps remove unused CSS. To further optimize, you can use tools like PurgeCSS or Tailwind CSS to eliminate unused styles in the final product.
Using Tailwind CSS for optimization: If you're using Tailwind CSS, PurgeCSS automatically removes unused CSS classes during build, reducing CSS size.
// tailwind.config.js module.exports = { purge: ['./pages/**/*.tsx', './components/**/*.tsx'], darkMode: false, theme: { extend: {} }, variants: { extend: {} }, plugins: [] };
5. Caching and CDN
To increase page load speed, you can use caching and CDNs to serve static assets like images, CSS, and JavaScript. Next.js integrates well with platforms like Vercel and Netlify, which serve your app from edge locations closest to users.
How to use:
You can configure caching in Next.js through next.config.js
to define appropriate caching strategies for your app’s resources.
module.exports = { async headers() { return [ { source: '/(.*).(js|css|png|jpg|jpeg|svg|gif|webp|ico)', headers: [ { key: 'Cache-Control', value: 'public, max-age=31536000, immutable', }, ], }, ]; }, };
6. Improve SEO with Server-Side Rendering (SSR)
If your application requires better SEO, you can use Server-Side Rendering (SSR) in Next.js to render content on the server before sending it to the client. This helps search engines index your content more effectively.
// pages/index.tsx import { GetServerSideProps } from 'next'; export const getServerSideProps: GetServerSideProps = async () => { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data } }; };
Conclusion
Performance optimization in Next.js not only enhances the user experience but also contributes to SEO growth and application scalability. By leveraging features like SSG, image optimization, dynamic imports, and caching, you can build fast and efficient Next.js applications.