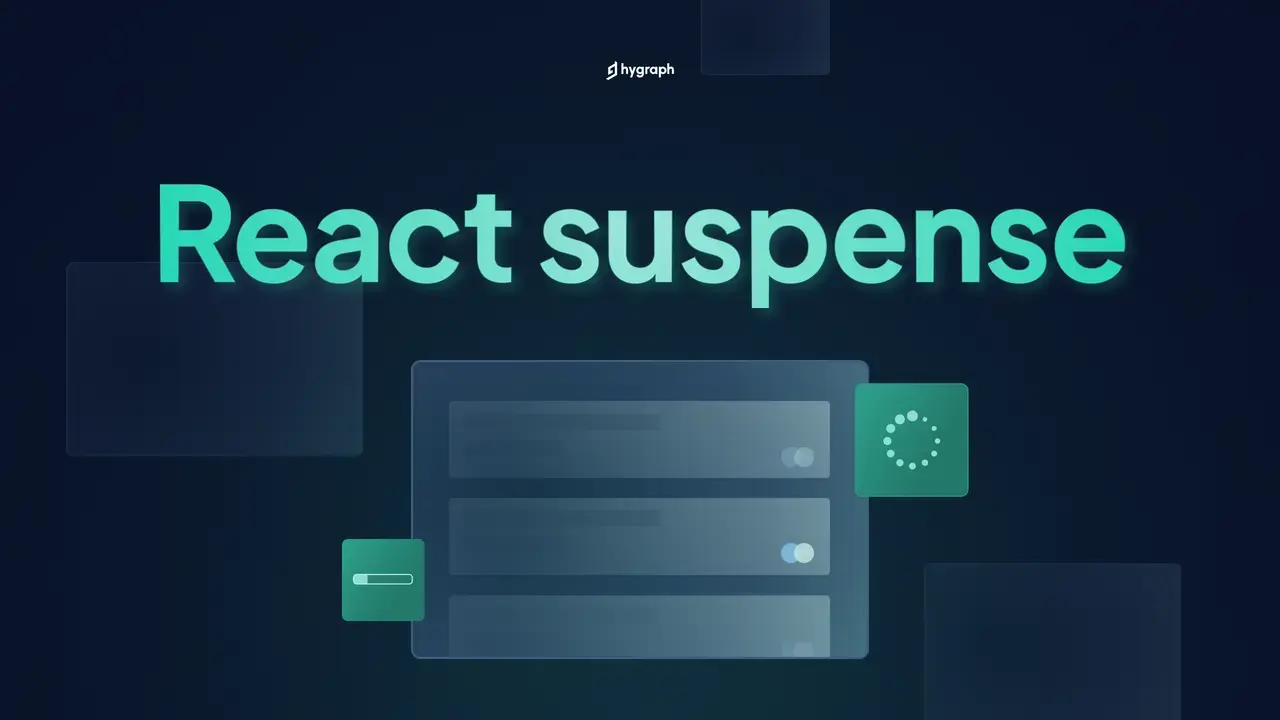
February 12, 2025
React Suspense: Chìa khóa cho trải nghiệm người dùng mượt mà
Tìm hiểu về React Suspense và cách nó giúp tối ưu trải nghiệm người dùng.
React Suspense là gì?
React Suspense là một cơ chế cho phép React "tạm dừng" việc render một thành phần UI cho đến khi dữ liệu cần thiết sẵn sàng. Nó giúp giảm thiểu việc hiển thị giao diện chưa hoàn thiện và tăng tốc tải trang.
1. Tại sao Suspense quan trọng?
Khi nội dung phụ thuộc vào dữ liệu fetch từ API, trang web có thể hiển thị giao diện chưa hoàn chỉnh, gây trải nghiệm khó chịu. Suspense giúp tạo ra "loading states" mệt mà và giữ UI trông tự nhiên hơn.
2. Cách sử dụng Suspense trong React
Suspense hoạt động tốt nhất khi kết hợp với React.lazy để tải component một cách lazy.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
export default function Page() {
return (
<Suspense fallback={<p>Loading...</p>}>
<LazyComponent />
</Suspense>
);
}
Giải thích:
lazy()
giúp load component chỉ khi cần.Suspense
bao quanh component và hiển thị UI fallback khi chưa load xong.
3. Suspense và Data Fetching
Suspense không chỉ giúp tải component mà còn hỗ trợ fetch dữ liệu bên trong Server Components (Next.js 13+).
export default async function Page() {
const data = await fetchData();
return (
<Suspense fallback={<p>Loading data...</p>}>
<DataComponent data={data} />
</Suspense>
);
}
Lưu ý: React Suspense chưa hỗ trợ fetch API trên client (phải kết hợp với React Cache hoặc Relay).
4. Kết hợp Suspense với React Server Components
Trong Next.js, Suspense hoạt động cực kỳ hiệu quả khi render bên server, giúp cải thiện performance.
import { Suspense } from 'react';
import UserProfile from './UserProfile';
export default function Page() {
return (
<Suspense fallback={<p>Loading profile...</p>}>
<UserProfile />
</Suspense>
);
}
5. Tổng kết: Khi nào nên dùng Suspense?
- Khi load component theo nhu cầu (Lazy Loading).
- Khi fetch dữ liệu trong Server Components.
- Khi muốn tối ưu trải nghiệm người dùng với fallback UI.
React Suspense giúp tối ưu hiển thị UI và giảm thiểu trạng thái "trắng" khi tải trang. Sử dụng nó đúng cách sẽ tăng tốc và giừm mượt cho ứng dụng React của bạn!
React Suspense: The Key to a Smooth User Experience
Learn about React Suspense and how it helps optimize user experience.
What is React Suspense?
React Suspense is a mechanism that allows React to "pause" rendering a UI component until the necessary data is ready. It helps reduce the display of incomplete UI and speeds up page loading.
1. Why is Suspense important?
When content depends on data fetched from an API, the website might display incomplete UI, which can lead to a poor user experience. Suspense helps create "loading states" that feel natural and keep the UI looking seamless.
2. How to use Suspense in React
Suspense works best when combined with React.lazy to lazy-load components.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
export default function Page() {
return (
<Suspense fallback={<p>Loading...</p>}>
<LazyComponent />
</Suspense>
);
}
Explanation:
lazy()
loads a component only when needed.Suspense
wraps the component and displays a fallback UI until the component is loaded.
3. Suspense and Data Fetching
Suspense not only helps with lazy-loading components but also supports fetching data inside Server Components (Next.js 13+).
export default async function Page() {
const data = await fetchData();
return (
<Suspense fallback={<p>Loading data...</p>}>
<DataComponent data={data} />
</Suspense>
);
}
Note: React Suspense does not yet support the fetch API on the client (it must be combined with React Cache or Relay).
4. Combining Suspense with React Server Components
In Next.js, Suspense works extremely well when rendering on the server, improving performance.
import { Suspense } from 'react';
import UserProfile from './UserProfile';
export default function Page() {
return (
<Suspense fallback={<p>Loading profile...</p>}>
<UserProfile />
</Suspense>
);
}
5. Summary: When should you use Suspense?
- When loading components on-demand (Lazy Loading).
- When fetching data in Server Components.
- When you want to optimize the user experience with fallback UI.
React Suspense helps optimize UI rendering and minimize "blank" states during page load. Using it correctly will speed up and smooth out your React application!