April 2, 2025
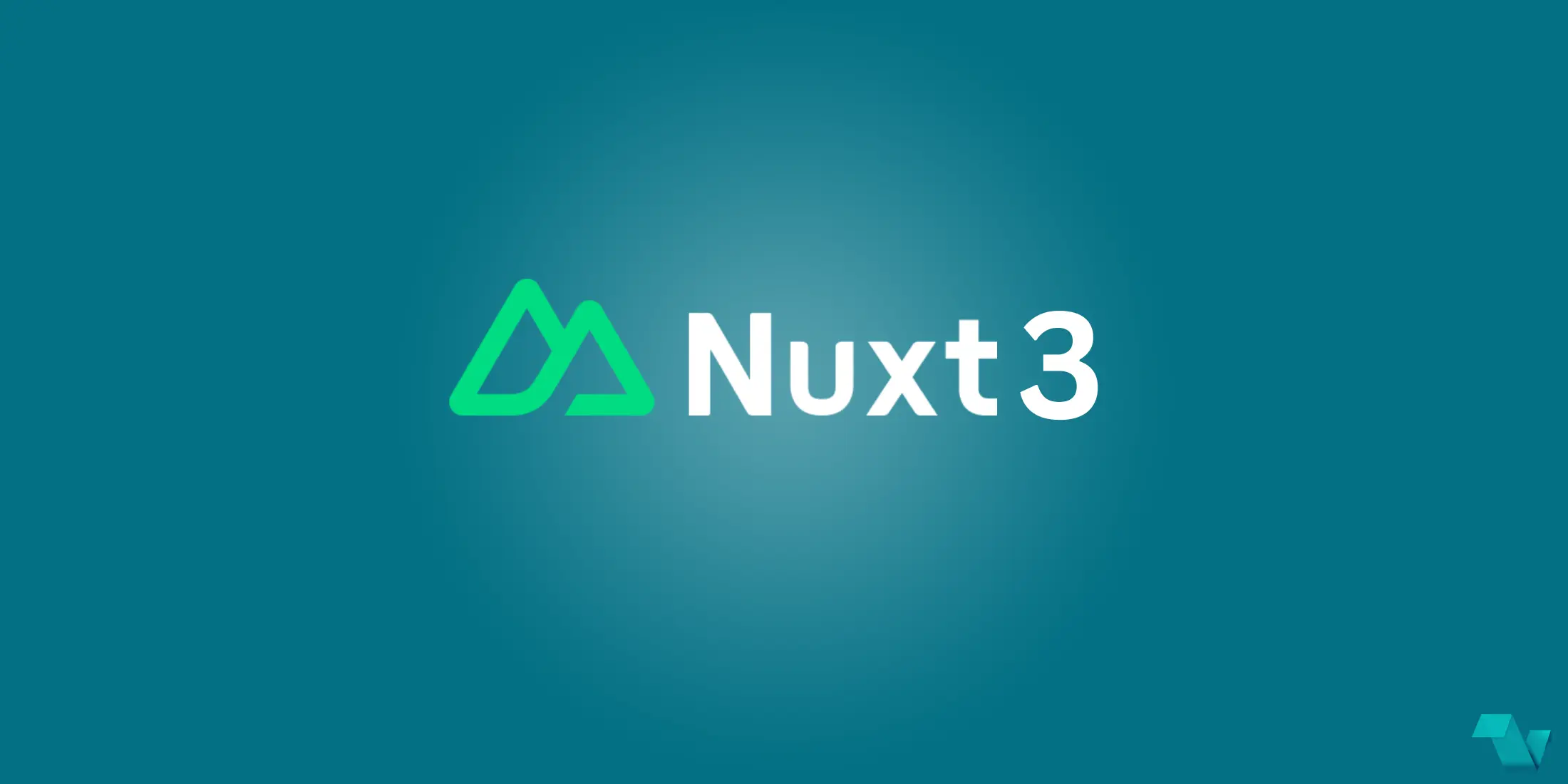
Nuxt 3: Những cải tiến và cách tận dụng tối đa
Tìm hiểu về những cải tiến trong Nuxt 3 và cách áp dụng chúng để xây dựng ứng dụng tối ưu.
Nuxt 3 có gì mới?
Nuxt 3 mang đến nhiều cải tiến quan trọng về hiệu suất, trải nghiệm lập trình viên và khả năng mở rộng ứng dụng. Dưới đây là một số điểm nổi bật:
- Hiệu suất nhanh hơn: Nhờ Vite làm trình build mặc định, tốc độ phát triển và bundle giảm đáng kể.
- Nitro Engine: Một server engine mạnh mẽ giúp Nuxt hoạt động tốt hơn trên nhiều môi trường như Serverless, Edge, hoặc truyền thống.
- Vue 3 & Composition API: Hỗ trợ tốt hơn cho Reactivity và TypeScript.
- Hỗ trợ Native TypeScript: Không cần cấu hình phức tạp để dùng TypeScript.
- File-based Routing: Cải tiến so với Nuxt 2 giúp định tuyến dễ dàng hơn.
1. Cách cài đặt Nuxt 3
Bạn có thể khởi tạo một dự án Nuxt 3 mới bằng cách sử dụng nuxi
:
npx nuxi init my-nuxt-app cd my-nuxt-app npm install npm run dev
Câu lệnh trên giúp tạo một ứng dụng Nuxt 3 nhanh chóng với các cài đặt mặc định.
2. Cải tiến trong cấu trúc thư mục
Nuxt 3 sử dụng cách tổ chức thư mục linh hoạt hơn, tập trung vào tự động hoá và giảm boilerplate code.
/pages/
→ Routing tự động./composables/
→ Chứa các hooks sử dụng lại./server/
→ Chứa API endpoints cho Backend trong cùng dự án./app.vue
→ Entry point chính của ứng dụng.
Ví dụ về API đơn giản trong Nuxt 3:
// server/api/hello.ts
export default defineEventHandler(() => {
return { message: 'Hello from Nuxt 3 API!' };
});
3. Sử dụng Nitro Engine để tối ưu server
Nitro là nền tảng mới trong Nuxt 3 giúp cải thiện hiệu suất server-side rendering (SSR) và hỗ trợ nhiều môi trường triển khai như Vercel, Netlify, Cloudflare Workers.
Ví dụ tạo API với Nitro:
export default defineEventHandler(async (event) => {
const data = await fetch('https://jsonplaceholder.typicode.com/posts');
return await data.json();
});
4. Kết hợp Nuxt 3 với Pinia để quản lý trạng thái
Pinia là thư viện quản lý trạng thái chính thức cho Vue 3 và hoạt động mượt mà với Nuxt 3.
Cài đặt Pinia:
npm install pinia
Tạo store đơn giản:
// store/counter.ts
import { defineStore } from 'pinia';
export const useCounterStore = defineStore('counter', {
state: () => ({ count: 0 }),
actions: {
increment() {
this.count++;
}
}
});
Dùng store trong component:
<script setup> import { useCounterStore } from '@/store/counter'; const counter = useCounterStore(); </script> <template> <button @click="counter.increment()">Count: {{ counter.count }}</button> </template>
5. Triển khai Nuxt 3 lên Vercel
Nhờ Nitro Engine, Nuxt 3 có thể triển khai dễ dàng trên các nền tảng serverless như Vercel.
Cài đặt Vercel CLI:
npm install -g vercel vercel
Sau đó, làm theo hướng dẫn để hoàn tất triển khai.
6. Tổng kết
Nuxt 3 mang lại nhiều cải tiến quan trọng:
✅ Build nhanh hơn nhờ Vite.
✅ SSR mạnh mẽ hơn với Nitro Engine.
✅ Hỗ trợ tốt Vue 3 & TypeScript.
✅ Quản lý trạng thái dễ dàng với Pinia.
Nếu bạn đang xây dựng một ứng dụng Vue hiện đại, hãy tận dụng Nuxt 3 để tối ưu hiệu suất và trải nghiệm lập trình!
Nuxt 3: Improvements and How to Make the Most of Them
Learn about the improvements in Nuxt 3 and how to apply them to build optimized applications.
What's New in Nuxt 3?
Nuxt 3 brings several important improvements in performance, developer experience, and application scalability. Here are some highlights:
- Faster performance: With Vite as the default build tool, development speed and bundle size are significantly reduced.
- Nitro Engine: A powerful server engine that helps Nuxt perform better across various environments like Serverless, Edge, or traditional servers.
- Vue 3 & Composition API: Better support for Reactivity and TypeScript.
- Native TypeScript support: No need for complex configuration to use TypeScript.
- File-based Routing: Improvements over Nuxt 2 make routing easier.
1. How to Install Nuxt 3
You can initialize a new Nuxt 3 project using nuxi
:
npx nuxi init my-nuxt-app cd my-nuxt-app npm install npm run dev
This command quickly creates a Nuxt 3 application with default settings.
2. Improvements in Folder Structure
Nuxt 3 uses a more flexible folder structure, focusing on automation and reducing boilerplate code.
/pages/
→ Automatic routing./composables/
→ Contains reusable hooks./server/
→ Contains API endpoints for the backend within the same project./app.vue
→ The main entry point of the app.
Example of a simple API in Nuxt 3:
// server/api/hello.ts
export default defineEventHandler(() => {
return { message: 'Hello from Nuxt 3 API!' };
});
3. Using Nitro Engine for Server Optimization
Nitro is a new platform in Nuxt 3 that improves server-side rendering (SSR) performance and supports various deployment environments like Vercel, Netlify, and Cloudflare Workers.
Example of creating an API with Nitro:
export default defineEventHandler(async (event) => {
const data = await fetch('https://jsonplaceholder.typicode.com/posts');
return await data.json();
});
4. Combining Nuxt 3 with Pinia for State Management
Pinia is the official state management library for Vue 3 and works seamlessly with Nuxt 3.
Install Pinia:
npm install pinia
Create a simple store:
// store/counter.ts
import { defineStore } from 'pinia';
export const useCounterStore = defineStore('counter', {
state: () => ({ count: 0 }),
actions: {
increment() {
this.count++;
}
}
});
Use the store in a component:
<script setup> import { useCounterStore } from '@/store/counter'; const counter = useCounterStore(); </script> <template> <button @click="counter.increment()">Count: {{ counter.count }}</button> </template>
5. Deploying Nuxt 3 on Vercel
Thanks to Nitro Engine, Nuxt 3 can be easily deployed on serverless platforms like Vercel.
Install Vercel CLI:
npm install -g vercel vercel
Then, follow the prompts to complete the deployment.
6. Summary
Nuxt 3 brings many important improvements:
✅ Faster builds thanks to Vite.
✅ Stronger SSR with Nitro Engine.
✅ Better support for Vue 3 & TypeScript.
✅ Easier state management with Pinia.
If you're building a modern Vue application, take advantage of Nuxt 3 to optimize performance and developer experience!