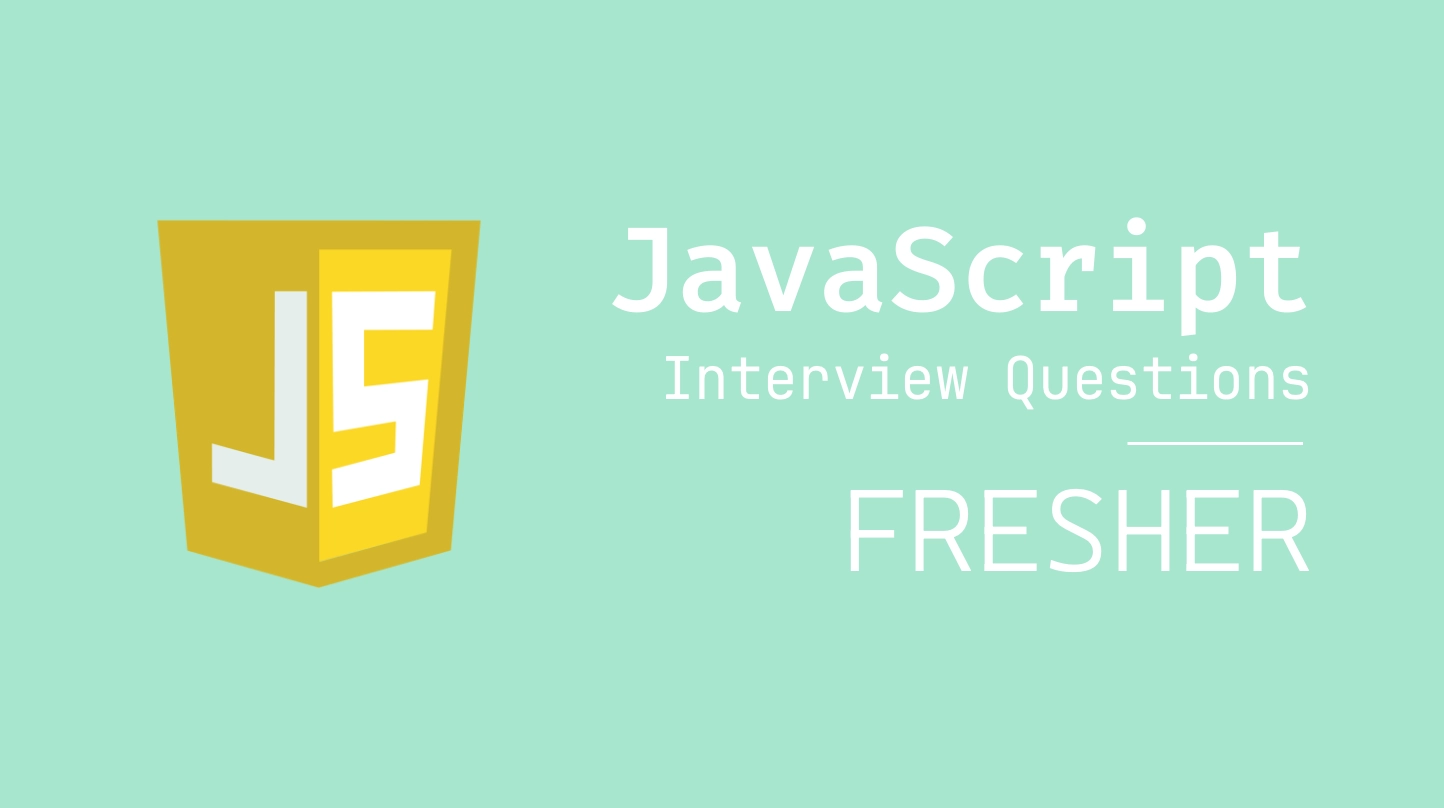
March 21, 2025
Câu hỏi phỏng vấn JavaScript cho Fresher
Tuyển tập các câu hỏi phỏng vấn JavaScript cơ bản dành cho Fresher, kèm giải thích dễ hiểu và ví dụ minh hoạ.
Q: var
, let
, const
khác nhau gì?
A: var
có phạm vi theo function, còn let
và const
có phạm vi theo block ({}
), giúp tránh lỗi khi khai báo trong vòng lặp hoặc if. let
cho phép gán lại giá trị, còn const
thì không. Ngoài ra, var
có hoisting – được kéo lên đầu scope nhưng không khởi tạo.
if (true) {
var a = 1;
let b = 2;
}
console.log(a); // 1
console.log(b); // ReferenceError
Q: ==
và ===
khác nhau gì?
A: ==
là so sánh lỏng (loose equality), có thể tự động ép kiểu. ===
là so sánh nghiêm ngặt (strict equality), yêu cầu cùng kiểu dữ liệu và giá trị. Dùng ===
giúp tránh các lỗi logic do ép kiểu ngầm.
0 == false // true 0 === false // false
Q: null
và undefined
khác gì nhau?
A: undefined
là giá trị mặc định khi biến chưa được gán. null
là giá trị được gán có chủ đích để biểu thị “không có gì”. Về bản chất, cả hai đều “falsy” nhưng khác ngữ nghĩa.
Q: JS có những kiểu dữ liệu nào?
A: JavaScript có hai loại kiểu dữ liệu: primitive và object. Primitive gồm: string
, number
, boolean
, null
, undefined
, symbol
, bigint
. Mọi thứ còn lại (array, function, date, ...) là object.
Q: typeof null
là gì? Sao lại vậy?
A: typeof null
trả về "object"
, do một lỗi trong design ban đầu của JavaScript. Mặc dù null
là primitive, nhưng typeof
vẫn trả về object
. Đây là một “bug lịch sử”.
Q: Làm sao kiểm tra một biến có phải là mảng?
A: Dùng Array.isArray(value)
để kiểm tra chính xác. Tránh dùng typeof
vì typeof []
trả về "object"
.
Array.isArray([1, 2, 3]) // true
Array.isArray("abc") // false
Q: Làm sao ép kiểu string thành number?
A: Có nhiều cách: Number(str)
, parseInt(str)
, parseFloat(str)
, hoặc đơn giản là dùng dấu +
. Tuy nhiên, nên kiểm tra kỹ input để tránh trả về NaN
.
+"42" // 42
parseInt("42px") // 42
Number("42px") // NaN
Q: NaN
là gì và làm sao kiểm tra?
A: NaN
viết tắt “Not a Number”, là kết quả của phép tính không hợp lệ. Dùng Number.isNaN(value)
để kiểm tra chính xác, không dùng ==
vì NaN !== NaN
.
Q: Hàm là gì trong JavaScript?
A: Là một khối mã có thể tái sử dụng. JS hỗ trợ khai báo qua function
, function expression
, arrow function
. Arrow function không có this
riêng nên thường dùng trong context đơn giản.
Q: Sự khác biệt giữa null
, {}
và []
là gì?
A: null
là giá trị rỗng có chủ đích. {}
là object rỗng. []
là mảng rỗng. Chúng có vai trò và cách dùng khác nhau tùy vào dữ liệu bạn muốn lưu.
Q: Tại sao [] + []
lại ra chuỗi rỗng?
A: Vì khi dùng toán tử +
với mảng, JS sẽ ép mảng thành chuỗi, nên [] + []
trở thành "" + ""
= ""
.
Q: Template string là gì?
A: Là cách viết chuỗi dễ đọc, hỗ trợ chèn biến bằng ${}
. Sử dụng dấu backtick `
thay vì nháy đơn hay nháy kép.
const name = "Vũ"; console.log(`Xin chào ${name}`); // Xin chào Vũ
Q: Falsy values là gì?
A: Là những giá trị được convert thành false
trong ngữ cảnh boolean. Bao gồm: false
, 0
, ""
, null
, undefined
, NaN
.
Q: Có bao nhiêu cách khai báo biến?
A: Có 3 cách: var
(cũ, ít dùng), let
(thay thế var
), const
(không cho gán lại, dùng cho hằng số).
Q: Tại sao nên dùng const
nhiều hơn let
?
A: Vì const
giúp code an toàn hơn, tránh việc vô tình gán lại biến. Tuy nhiên, const
chỉ đảm bảo tham chiếu không đổi, object/mảng bên trong vẫn có thể thay đổi.
Q: Làm sao lặp qua mảng?
A: Có nhiều cách: for
, for...of
, forEach
, map
, filter
, reduce
. Mỗi cái có mục đích khác nhau, forEach
để thao tác, map
để tạo mảng mới, filter
để lọc,…
Q: typeof
có thể dùng kiểm tra mọi kiểu dữ liệu không?
A: Không hoàn toàn. Ví dụ typeof null
là "object"
, typeof []
cũng là "object"
. Để kiểm tra chính xác, nên dùng Object.prototype.toString.call(value)
.
Q: Làm sao sao chép mảng hoặc object?
A: Với mảng, dùng [...]
hoặc array.slice()
. Với object, dùng {...obj}
hoặc Object.assign()
. Tránh gán trực tiếp vì sẽ là tham chiếu.
const original = [1, 2];
const copy = [...original];
Q: JS là ngôn ngữ đồng bộ hay bất đồng bộ?
A: JavaScript là ngôn ngữ đơn luồng (single-threaded), nhưng có thể xử lý bất đồng bộ thông qua event loop, callback, Promise và async/await. Điều này giúp giao diện không bị "đơ" khi xử lý dữ liệu lâu.
Q: Sự khác nhau giữa function declaration
và function expression
là gì?
A: function declaration
được hoisting – bạn có thể gọi hàm trước khi khai báo. Trong khi đó, function expression
(gán hàm vào biến) không được hoisting, nên phải khai báo trước khi dùng.
sayHello(); // OK
function sayHello() {
console.log("Hi!");
}
// -
greet(); // Error: greet is not a function
const greet = function () {
console.log("Hello!");
};
Q: console.log(typeof NaN)
in ra gì?
A: "number"
. Mặc dù NaN
có nghĩa là “Not a Number”, kiểu dữ liệu của nó vẫn là number
. Đây là một đặc điểm kỳ lạ của JavaScript.
Q: Hàm parseInt("08")
trả về gì?
A: Trả về 8
. Nhưng nếu bạn không truyền radix
(cơ số), trong môi trường cũ, "08"
có thể bị hiểu là số hệ 8 và trả về 0
. Vì vậy nên luôn truyền cơ số: parseInt("08", 10)
.
Q: Toán tử typeof
với function trả về gì?
A: Trả về "function"
. Đây là cách duy nhất typeof
trả về cụ thể hơn "object"
, giúp phân biệt được function với object thông thường.
Q: Sự khác nhau giữa for...in
và for...of
là gì?
A: for...in
dùng để duyệt qua các key (tên thuộc tính) của object. for...of
dùng để duyệt qua các giá trị trong iterable (như array, string,...).
const arr = ["a", "b"]; for (const i in arr) console.log(i); // 0, 1 for (const v of arr) console.log(v); // "a", "b"
Q: Khi nào dùng break
và continue
?
A: break
thoát khỏi vòng lặp hoàn toàn. continue
bỏ qua vòng lặp hiện tại và tiếp tục vòng lặp tiếp theo. Dùng để điều khiển luồng trong vòng lặp.
Q: isNaN("123")
trả về gì?
A: Trả về false
vì "123"
có thể ép kiểu thành số. Tuy nhiên, nên dùng Number.isNaN()
để kiểm tra chính xác hơn vì isNaN()
cũ có thể bị ép kiểu sai.
Q: Sự khác nhau giữa setTimeout(fn, 0)
và gọi fn()
trực tiếp là gì?
A: fn()
chạy ngay lập tức. Còn setTimeout(fn, 0)
đợi call stack rỗng rồi mới chạy, nên thường dùng để đẩy hàm vào cuối vòng event loop.
Q: Arrow function có this
không?
A: Không. Arrow function không có this
riêng – nó kế thừa this
từ scope bên ngoài. Điều này hữu ích khi dùng trong callback để tránh bị mất this
.
Q: Có thể khai báo 2 biến trùng tên bằng var
trong cùng 1 scope không?
A: Có. JavaScript cho phép điều này với var
do hoisting, nhưng sẽ gây lỗi logic. let
và const
thì không cho phép khai báo trùng tên trong cùng scope.
JavaScript Interview Questions for Fresher
A collection of basic JavaScript interview questions for Fresher, with clear explanations and illustrative examples.
Q: What's the difference between var
, let
, and const
?
A: var
is function-scoped, while let
and const
are block-scoped ({}
), which helps avoid issues in loops or conditionals. let
allows reassignment, const
does not. Also, var
is hoisted – it's moved to the top of the scope but not initialized.
if (true) {
var a = 1;
let b = 2;
}
console.log(a); // 1
console.log(b); // ReferenceError
Q: What's the difference between ==
and ===
?
A: ==
is loose equality and allows type coercion. ===
is strict equality and requires both value and type to be the same. Using ===
helps avoid unexpected bugs caused by type coercion.
0 == false // true 0 === false // false
Q: What's the difference between null
and undefined
?
A: undefined
is the default value of uninitialized variables. null
is intentionally assigned to indicate "nothing." Both are falsy but have different meanings.
Q: What data types are there in JavaScript?
A: JavaScript has two main types: primitive and object. Primitive types include: string
, number
, boolean
, null
, undefined
, symbol
, bigint
. Everything else (arrays, functions, dates, etc.) is of type object.
Q: What's typeof null
? Why?
A: typeof null
returns "object"
due to a historical bug in JavaScript's design. Although null
is a primitive, it returns "object"
.
Q: How do you check if a variable is an array?
A: Use Array.isArray(value)
for accurate checking. Avoid using typeof
because typeof []
returns "object"
.
Array.isArray([1, 2, 3]) // true
Array.isArray("abc") // false
Q: How do you convert a string to a number?
A: Several ways: Number(str)
, parseInt(str)
, parseFloat(str)
, or simply +str
. However, always validate input to avoid returning NaN
.
+"42" // 42
parseInt("42px") // 42
Number("42px") // NaN
Q: What is NaN
and how do you check it?
A: NaN
stands for "Not a Number", returned from invalid math operations. Use Number.isNaN(value)
for accurate checking. Don't use ==
because NaN !== NaN
.
Q: What is a function in JavaScript?
A: A reusable block of code. You can define functions via function
, function expression
, or arrow function
. Arrow functions don’t have their own this
, which is useful in simple contexts.
Q: What's the difference between null
, {}
, and []
?
A: null
means intentional emptiness. {}
is an empty object. []
is an empty array. They serve different purposes depending on the data type.
Q: Why does [] + []
result in an empty string?
A: Because the +
operator coerces arrays into strings. So [] + []
becomes "" + ""
= ""
.
Q: What are template strings?
A: A cleaner way to write strings with embedded variables using ${}
. Use backticks `
instead of quotes.
const name = "Vũ"; console.log(`Hello ${name}`); // Hello Vũ
Q: What are falsy values?
A: Values that convert to false
in a boolean context. These include: false
, 0
, ""
, null
, undefined
, NaN
.
Q: How many ways to declare a variable?
A: Three: var
(old, rarely used), let
(modern), const
(immutable reference, preferred for constants).
Q: Why use const
more than let
?
A: const
makes code safer by preventing accidental reassignment. However, it only ensures the reference is constant; the contents (like arrays/objects) can still change.
Q: How to loop through an array?
A: Many ways: for
, for...of
, forEach
, map
, filter
, reduce
. Use each depending on need – forEach
for actions, map
for transforming, filter
for filtering, etc.
Q: Can typeof
check all data types?
A: Not entirely. For example, typeof null
is "object"
and typeof []
is also "object"
. For accurate checks, use Object.prototype.toString.call(value)
.
Q: How to copy arrays or objects?
A: For arrays: use [...]
or array.slice()
. For objects: use {...obj}
or Object.assign()
. Avoid direct assignment as it creates references.
const original = [1, 2];
const copy = [...original];
Q: Is JavaScript synchronous or asynchronous?
A: JavaScript is single-threaded but supports asynchronous behavior via the event loop, callbacks, Promises, and async/await. This prevents the UI from freezing during long operations.
Here are a few additional Fresher-level questions and answers – still simple, easy to understand, and with examples where needed.
Q: What's the difference between function declaration
and function expression
?
A: function declaration
is hoisted – you can call the function before it’s declared. function expression
(assigned to a variable) is not hoisted, so it must be defined before use.
sayHello(); // OK
function sayHello() {
console.log("Hi!");
}
// -
greet(); // Error: greet is not a function
const greet = function () {
console.log("Hello!");
};
Q: What does console.log(typeof NaN)
output?
A: "number"
. Even though NaN
means “Not a Number”, its type is still number
. This is one of JavaScript’s quirks.
Q: What does parseInt("08")
return?
A: 8
. However, in older environments, if you don’t pass a radix, "08"
may be interpreted as octal and return 0
. So always pass the radix: parseInt("08", 10)
.
Q: What does typeof
return for functions?
A: "function"
. It's the only case where typeof
returns something more specific than "object"
, helping distinguish functions from regular objects.
Q: What's the difference between for...in
and for...of
?
A: for...in
iterates over object keys (property names). for...of
iterates over iterable values (arrays, strings, etc.).
const arr = ["a", "b"]; for (const i in arr) console.log(i); // 0, 1 for (const v of arr) console.log(v); // "a", "b"
Q: When to use break
and continue
?
A: break
exits the loop completely. continue
skips the current loop iteration and proceeds to the next. Useful for controlling loop flow.
Q: What does isNaN("123")
return?
A: false
– because "123"
can be coerced into a number. Prefer Number.isNaN()
for reliable checks, as isNaN()
can mislead due to coercion.
Q: Difference between setTimeout(fn, 0)
and calling fn()
directly?
A: fn()
runs immediately. setTimeout(fn, 0)
delays execution until the call stack is clear – it pushes the function to the end of the event loop queue.
Q: Do arrow functions have their own this
?
A: No. Arrow functions inherit this
from the outer scope. This is useful in callbacks to avoid losing context.
Q: Can you declare two var
variables with the same name in the same scope?
A: Yes. JavaScript allows it due to var
hoisting, but it can lead to logic errors. let
and const
do not allow duplicate declarations in the same scope.